Introduction:
In the world of Android development, designing robust and maintainable applications is paramount. To achieve this, developers often turn to architectural patterns that promote separation of concerns and enhance code readability. One such pattern is the Model-View-ViewModel (MVVM) architecture. MVVM has gained popularity due to its ability to efficiently handle complex UI interactions while keeping the codebase organized. In this blog post, we will delve into the details of MVVM architecture and explore its key components and benefits.
What is MVVM?
MVVM is an architectural pattern that facilitates the separation of concerns between the user interface (View), the business logic and data (ViewModel), and the data storage or services (Model). It promotes a structured approach to building Android applications, allowing for better code maintainability, testability, and scalability.
Components of MVVM Architecture:
- Model: The Model represents the data and business logic of the application. It encapsulates data retrieval, manipulation, and storage operations. In Android, the Model can include classes that interact with databases, web services, or any other data sources.
- View: The View is responsible for rendering the user interface and displaying information to the user. It includes XML layout files, activity/fragment classes, and UI-related logic. Views are kept as lightweight as possible and should avoid containing business logic.
- ViewModel: The ViewModel acts as a bridge between the View and the Model. It exposes data and methods required by the View and provides a separation from the underlying business logic. The ViewModel retrieves data from the Model, prepares it for the View, and handles user interactions. It also allows for data transformation and formatting.
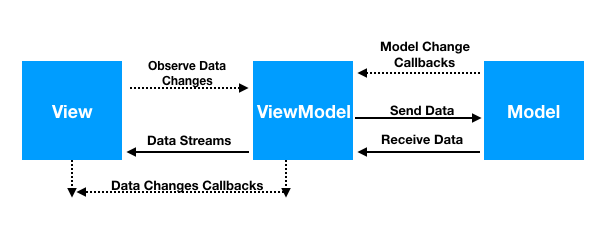
The Data Binding Library:
The Data Binding Library is a key component of MVVM architecture in Android. It simplifies the process of connecting UI components in the View directly to the data in the ViewModel. This eliminates the need for manual view updates and reduces boilerplate code. Data binding also allows for two-way data binding, where changes in the View automatically update the ViewModel and vice versa.
Implementing MVVM in Android:
- Setting Up Dependencies:
To implement MVVM, start by adding the necessary dependencies in your project’s build.gradle file. Include the Data Binding Library, ViewModel, and LiveData dependencies. - Creating the Model:
Define your data sources, repositories, and any necessary business logic classes. These components will handle data retrieval and manipulation. - Developing the ViewModel:
Create a ViewModel class for each View in your application. The ViewModel should expose LiveData objects that the View can observe to receive data updates. It should also include methods for handling user interactions and updating the Model. - Building the View:
Define the XML layout for your View and use data binding to connect UI components to the ViewModel. Bind the LiveData objects from the ViewModel to the corresponding UI elements, allowing them to be automatically updated when the data changes.
Advantages of MVVM:
- Separation of concerns: MVVM helps in maintaining a clear separation between the UI, business logic, and data, making the codebase more maintainable and testable.
- Testability: With MVVM, both the View and ViewModel can be tested independently using unit tests, as the ViewModel does not rely on the Android framework.
- Code reusability: MVVM promotes reusability by separating UI logic from the underlying data and business logic, enabling easier adaptation to different form factors and configurations.
- Improved collaboration: MVVM facilitates collaboration between designers and developers. Designers can work on the layout and UI independently, while developers focus on the ViewModel and business logic.
Conclusion:
The MVVM architecture is a powerful pattern for building Android applications. It enables clean separation of concerns, enhances testability, and promotes code reusability. By leveraging the Data Binding Library and following best practices, developers can create maintainable and scalable applications. Understanding and implementing MVVM will undoubtedly elevate your Android development skills and lead to more efficient and robust applications.