FCM (Firebase Cloud Messaging) is a cloud messaging platform developed by Google that enables developers to send notifications and messages to users across a variety of platforms, including Android, iOS, and web applications. FCM is the successor to Google Cloud Messaging (GCM) and is designed to be more reliable, scalable, and flexible than its predecessor. It allows developers to send messages to individual devices, groups of devices, or topics that devices have subscribed to, and supports a range of message types, including notification messages, data messages, and messages with both notification and data payloads. FCM also provides tools for monitoring message delivery, analyzing message statistics, and managing message topics and subscriptions.
1. Add Firebase Core
Before any Firebase services can be used, you must first install the firebase_core
plugin, which is responsible for connecting your application to Firebase.
Install the plugin by running the following command from the project root:
flutter pub add firebase_core
2. Initializing FlutterFire
To initialize flutterfire we need Firebase CLI. You can download it from here. Make sure you move this firebase-cli exe file inside your project’s root directory.
Once you download and install the CLI, open it and it it should look something like this,
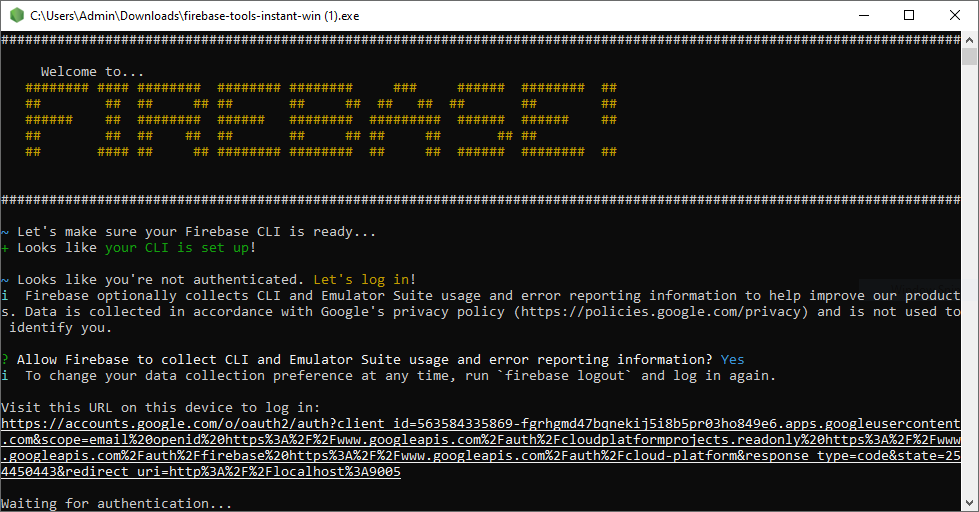
It will ask for “Allow Firebase to collect CLI and Emulator Suite usage and error reporting information? [y/n]”, here you need to allow this by pressing “y” and “enter”. Then it will open firebase on your browser and you have to login with your desired email address. Once you do this your CLI will be showing “Success! Logged in as {youremail@example.com}”
If your cli shows any JSON error, don’t worry. Just enter the command “firebase login” and you are good to go.
Now to test that the CLI is properly installed and accessing your account by listing your Firebase projects. Run the following command:
firebase projects:list
It should display all the firebase projects under your logged in account.
Now run this command to add firebase messaging
flutter pub add firebase_messaging
Now let’s initialize your firebase project with this flutter project
Run this command below,
firebase init
Now if it asks for confirmation hit yes and follow the next steps. In the next command it should ask you to select firebase feature, Just select the cloud function and hit enter [You can navigate using ‘arrow’ keys and select a feature with ‘space’ key]. After that it should ask you to choose an existing project or create a new one. You can select any of your choice. Now follow the commands until you see “Firebase initialization complete!” message.
3. App Setup
Now import the firebase cloud messaging in your main.dart file by adding this line,
import 'package:firebase_messaging/firebase_messaging.dart';
Now add these below codes in your main method,
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp(
options: DefaultFirebaseOptions.currentPlatform,
);
FirebaseMessaging messaging = FirebaseMessaging.instance;
messaging.getToken().then((value) {
String? token = value;
print("Device id: $token");
});
NotificationSettings settings = await messaging.requestPermission(
alert: true,
announcement: false,
badge: true,
carPlay: false,
criticalAlert: false,
provisional: false,
sound: true,
);
print('User granted permission: ${settings.authorizationStatus}');
FirebaseMessaging.onMessage.listen((RemoteMessage message) {
print('Got a message whilst in the foreground!');
print('Message data: ${message.data}');
RemoteNotification notification = message.notification!;
AndroidNotification? android = message.notification?.android;
if (message.notification != null) {
print('Message also contained a notification: ${message.notification}');
}
});
FirebaseMessaging.onBackgroundMessage(_firebaseMessagingBackgroundHandler);
}
Future<void> _firebaseMessagingBackgroundHandler(RemoteMessage message) async {
await Firebase.initializeApp();
print("Handling a background message: ${message.messageId}");
print("Message: ${message.data}");
}
Now rebuild your app with the following command,
flutter run
4. Send Notification from Firebase Console
Now just go to your firebase console from browser and send a notification to your app/project. You will receive the notification in real-time right in your app.