Introduction
When developing Android applications, understanding the lifecycle of activities and fragments is essential for building robust and responsive apps. The Android platform provides a well-defined lifecycle that governs the behavior and state management of these components. In this blog post, we will explore the intricacies of the Activity and Fragment lifecycle, discussing its phases, methods, and practical implications for Android developers.
The Activity Lifecycle
An Activity is a fundamental building block of an Android application, representing a single screen with a user interface. The Activity lifecycle consists of several distinct phases that occur as the user interacts with the app or system events take place. Let’s dive into the different stages of the Activity lifecycle:
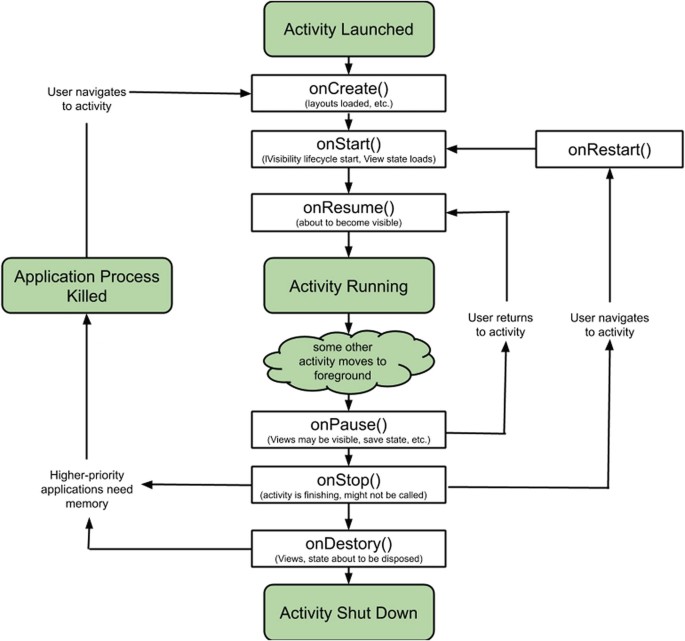
- onCreate(): This method is called when the activity is first created. It is where you typically initialize essential components, bind data, and set up the user interface.
- onStart(): This method is invoked when the activity becomes visible to the user, but it is not yet in the foreground. It is an excellent place to start animations or prepare resources that will be used in the onResume() method.
- onResume(): This method indicates that the activity is in the foreground and ready to interact with the user. It is the ideal spot to start or resume animations, register broadcast receivers, or acquire system resources.
- onPause(): This method is called when the activity loses focus but is still partially visible. It is crucial to pause ongoing operations, release resources, and save persistent data during this phase.
- onStop(): This method is invoked when the activity is no longer visible to the user. It is a suitable location to release resources that are not needed while the activity is not visible.
- onDestroy(): This method is called before the activity is destroyed. It is an excellent place to perform final cleanup, release system resources, and unregister any registered listeners or receivers.
Understanding the Fragment Lifecycle
Fragments represent reusable portions of the user interface within an Activity. They have their own lifecycle, which is closely related to the lifecycle of the hosting Activity. Let’s explore the different stages of the Fragment lifecycle:
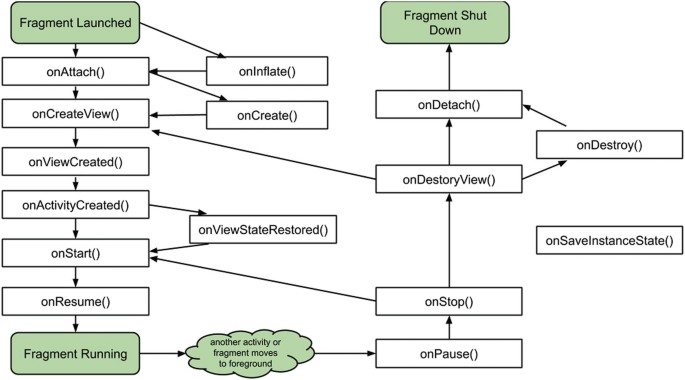
- onAttach(): This method is called when the fragment is attached to an Activity. It allows the fragment to initialize its communication with the hosting Activity.
- onCreate(): This method is similar to the Activity’s onCreate() method. It is where you initialize essential components and set up the fragment’s user interface.
- onCreateView(): This method is responsible for creating the fragment’s view hierarchy, usually by inflating a layout file. It is a suitable location to set up UI elements and bind data to them.
- onActivityCreated(): This method is called when the hosting Activity’s onCreate() method has completed. It is an excellent place to perform initialization that depends on the Activity’s state.
- onStart(), onResume(), onPause(), onStop(), and onDestroy(): These methods have the same semantics as the corresponding Activity lifecycle methods. They are called when the hosting Activity goes through these phases.
- onDetach(): This method is called when the fragment is no longer attached to an Activity. It allows the fragment to clean up any resources associated with the hosting Activity.
Leveraging the Lifecycle for Efficient Development
Understanding the Activity and Fragment lifecycle offers numerous benefits for Android developers:
- State Management: By leveraging the lifecycle methods, developers can save and restore the state of activities and fragments during configuration changes, such as device rotation or screen size adjustments.
- Resource Optimization: Knowing when to acquire and release resources ensures efficient memory usage and prevents memory leaks. For example, releasing resources in the onPause() or onStop() methods helps conserve system resources when the app is not in the foreground.
- User Experience: By appropriately managing the lifecycle, developers can create seamless user experiences. For instance,