Introduction:
Memory optimization plays a crucial role in developing high-performance Android applications. As smartphones become more powerful and feature-rich, optimizing memory usage becomes increasingly important to ensure smooth user experiences and efficient resource management. In this blog, we will explore various memory optimization techniques that can enhance the performance and efficiency of your Android apps.
1. Understand Android Memory Management:
Before delving into optimization techniques, it’s essential to understand how Android manages memory. Android uses a combination of techniques, including garbage collection (GC) and automatic memory allocation, to handle memory management. Android’s GC automatically reclaims memory by identifying and removing objects that are no longer in use. Familiarizing yourself with these concepts will provide a solid foundation for memory optimization.
2. Minimize Object Creation:
Excessive object creation can lead to increased memory consumption and frequent garbage collection cycles, impacting app performance. To minimize object creation, consider the following practices:
- Reuse objects: Instead of creating new objects, reuse existing ones whenever possible. This approach reduces memory overhead and improves performance.
- Use object pools: Implement object pooling to manage a pre-allocated set of objects. This technique avoids frequent object creation and destruction, thus optimizing memory usage.
3. Efficient Bitmap Handling:
Bitmaps are memory-intensive objects, especially when dealing with high-resolution images. To optimize bitmap handling:
- Scale down images: Resize images to an appropriate size based on the device’s screen resolution. This reduces memory consumption without compromising visual quality.
- Use the appropriate bitmap format: Choose bitmap formats that match the color depth requirements of your app. For example, if your app doesn’t require transparency, consider using RGB_565 instead of ARGB_8888, as it consumes less memory.
4. Memory Leaks Detection and Prevention:
Memory leaks occur when objects are unintentionally retained in memory, even when they are no longer needed. Over time, these leaks can accumulate and consume a significant amount of memory, leading to performance issues. To detect and prevent memory leaks:
- Use memory profiling tools: Android Studio provides memory profiling tools that can help identify memory leaks in your app. Analyze memory allocations and deallocations to identify potential leaks.
- Release resources appropriately: Ensure that you release resources such as files, database connections, and network connections when they are no longer needed. Failing to do so can result in memory leaks.
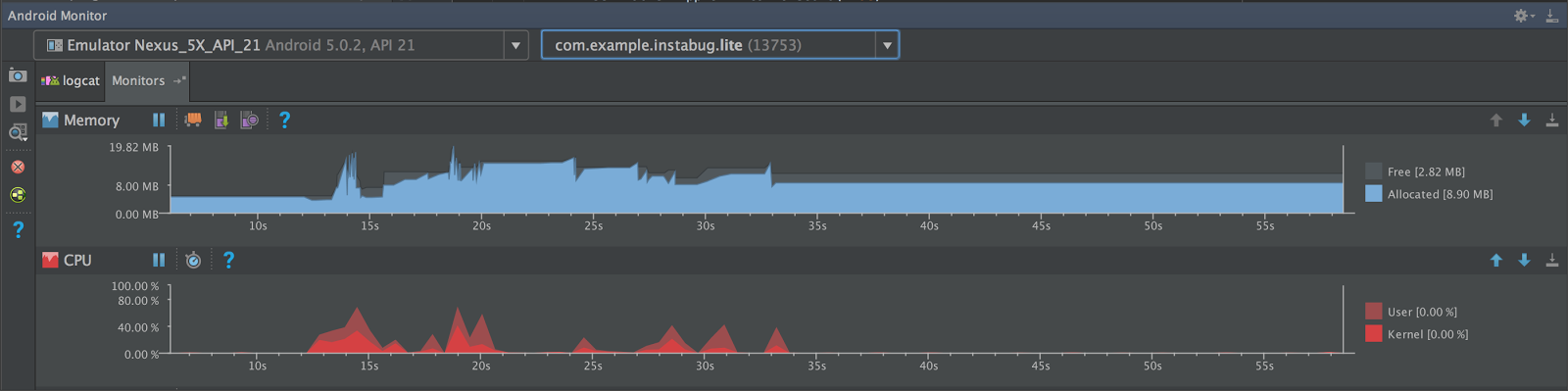
5. Implement Lazy Loading and Pagination:
Loading large data sets into memory at once can strain system resources and impact app performance. Implementing lazy loading and pagination techniques can significantly improve memory usage:
- Lazy loading: Load data only when it is required, instead of loading everything upfront. For example, when working with long lists, load data incrementally as the user scrolls through the list.
- Pagination: Divide large data sets into smaller chunks and load them as needed. This approach minimizes memory usage by loading only a portion of the data at a given time.
6. Optimize Background Processing:
Background tasks, such as network requests and data processing, can consume substantial memory if not handled efficiently. Consider the following approaches to optimize background processing:
- Use appropriate data structures: Choose the most efficient data structures for your background tasks. For example, if you need to store and process a large amount of data, consider using data structures like HashMaps or SparseArrays that offer efficient memory usage and retrieval.
- Limit parallel execution: Restrict the number of parallel background tasks to avoid excessive memory consumption. Use task queues or thread pools to manage task execution efficiently.
7. Use Memory Caching:
Memory caching helps reduce the load on system resources by storing frequently accessed data in memory. By caching data, you can retrieve it quickly without the need for expensive disk or network operations. Use memory caching libraries, such as LruCache or DiskLruCache, to implement efficient caching mechanisms in your app.
8. Optimize UI and View Handling:
The user interface (UI) is a critical component of any Android app, and inefficient UI design can impact memory usage. Consider the following tips to optimize UI and view handling:
- Avoid nested layouts: Deeply nested layouts can lead to inflated view hierarchies, resulting in increased memory usage. Simplify your UI structure by using efficient layouts like ConstraintLayout or RecyclerView.
- Use ViewHolder pattern: When working with RecyclerView, implement the ViewHolder pattern to recycle and reuse views efficiently, reducing memory overhead.
- Release references to views: Ensure that you release references to views when they are no longer needed. Holding onto view references can prevent the associated memory from being reclaimed.
Conclusion:
Memory optimization is crucial for developing high-performance Android apps. By implementing the techniques discussed in this blog, you can enhance your app’s efficiency, reduce memory consumption, and provide a smooth user experience. Remember to profile your app regularly to identify and address any memory-related issues. With careful attention to memory optimization, you can deliver exceptional Android applications that perform optimally on a wide range of devices.
Love it….
Hi there colleagues, how is all, and what you would
like to say on the topic of this paragraph, in my view its in fact remarkable for me.